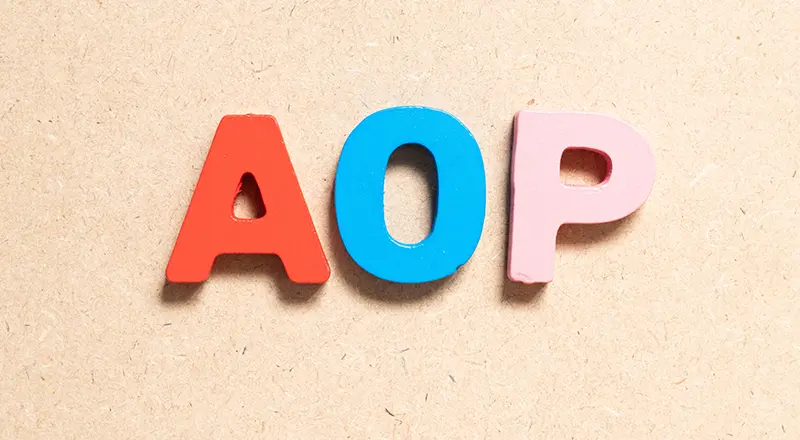
Aspect Oriented Programming is a methodology to separate cross cut code across different modules in a software system. All the cross cut code is moved to a separate module, thus increasing more modularity and bringing in ease of maintenance.
AOP has a number of benefits:
• Improves the performance of the application
• Manages Cross Cutting Concerns viz. logging, Execution time spent etc.
• Adheres to DRY (Don’t Repeat Yourself)
For example, for logging the code has to be written in each method, like method execution starts, ends and exception etc. This is nothing but repetition of code in all the methods.
Public void Submit ()
{
Try
{
Logger.Info(“Submit method starts”);
}
Catch
{
Logger.Info(“New Exception”);
}
Finally
{
Logger.Info(“Submit method completed”);
}
}
AOP helps in avoiding this repetition.
Types of AOP:
1. Interceptors
2. IL Code Weaving
Interceptors:
o Intercepts calls to class methods\properties
o Usually involves an Inversion of Control (IoC) Container
o No Post compilation changes to assemblies
IL Code Weaving:
o Runs after application compilation
o Post Process assemblies
Aspect Hook Locations:
• OnStart: Executed immediately prior to method call
• OnExit: Executed immediately after the method call runs
• OnSuccess: Executed immediately after the method call runs without throwing an exception
• OnError: Executed when a method throws exception
In this document, Let us see how the aspect-oriented programming is done using IL Code Weaving.
There are a few tools which allow youto perform IL Code Weaving.
1. Post Sharp
2. LOOM.NET
3. Wicca
Below implementation is done using Post Sharp.
Creating an Aspect:
– Implement a Base Class
– Attach the aspect
1. Create a Console Application for demonstrating the AOP using IL
Code Weaving.
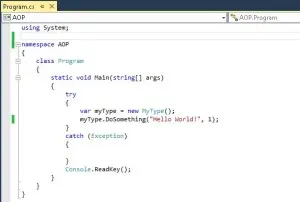
Output when the above code is executed:

2. Create another class called ‘LoggingAspect’ for logging the execution of the application. Add Post Sharp reference for this.
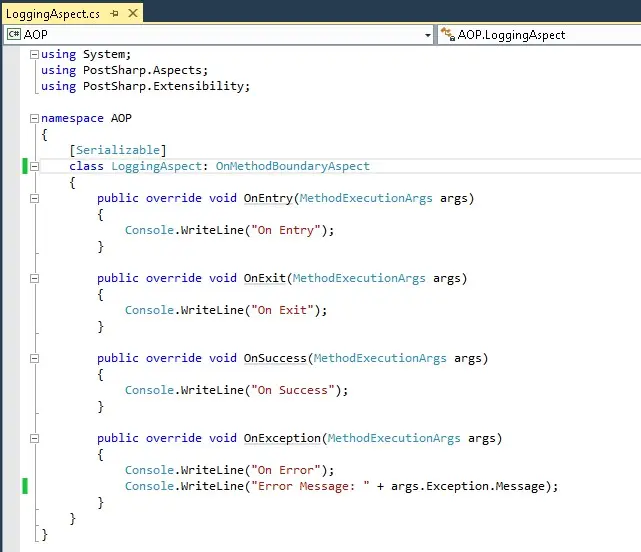
Inherit this class from the ‘OnMethodBoundaryAspect’ and add the attribute ‘Serializable’ to it.
3. Below is the output when the code is executed now. There’s no change in the output. Still AOP is not working as expected.

4. For the AOP to work as expected, the LoggingAspect needs to be attached to the underlying code.
5. Go to the MyType class and add the ‘LoggingAspect’ as an attribute to the method that needs to use the Aspect as shown below.
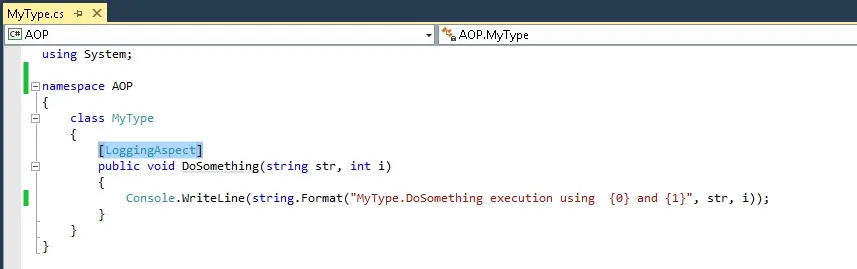
6. Run the application and observer the output. Logging is applied to the method.

7. In case, of any exception, the onException has to be logged. Add some code which throws an exception in the MyType class as below.
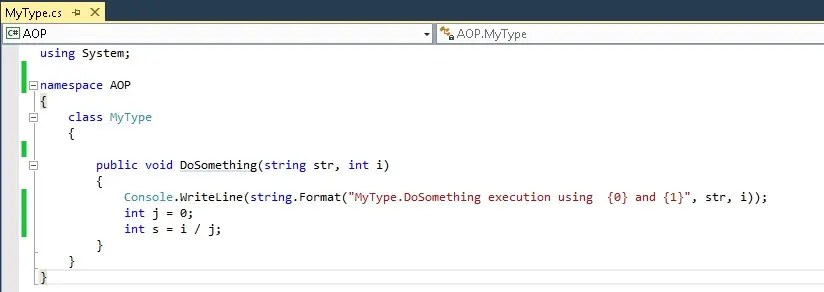
Output

The setting up of the aspect as an attribute can be done
– on a set of methods, by using the ‘LoggingAspect’ attribute on the required methods as shown above.
– on the whole application with the convention based approach. To apply the aspect on all the methods, update the assembly reference in the AssemblyInfo.cs of the Application as shown below
[assembly: LoggingAspect(AttributeTargetTypes = “AOP.*”)]
To attach the aspect only to the methods and not to the properties and constructors, we need to add the following attribute to the LoggingAspect.
[MulticastAttributeUsage (MulticastTargets.Method, TargetMemberAttributes=MulticastAttributes.Instance)]