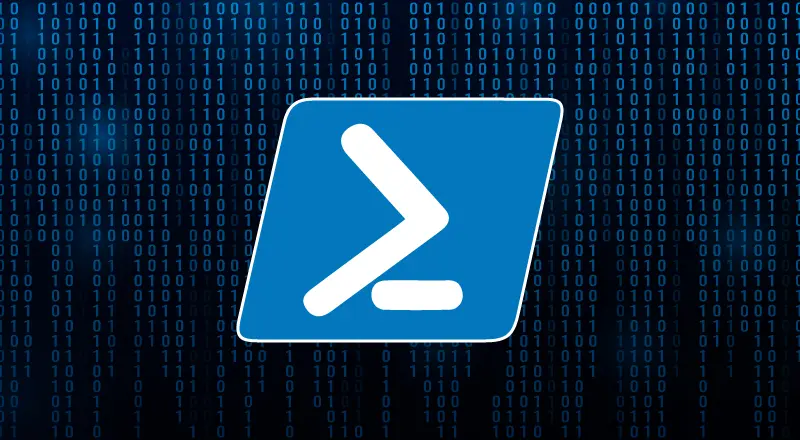
PowerShell is a cross-platform task automation solution made up of a command-line shell, a scripting language, and a configuration management framework. PowerShell runs on Windows, Linux, and macOS. In this article we will discuss about what is PowerShell, its features, cmdlet commands, data types and operator with examples.
What is PowerShell?
Microsoft designed PowerShell is an object-oriented scripting language and is designed mainly for the system administrators. It helps to control & automate the administration of the Window OS and other applications. It combines the flexibility of scripting, command-line speed, and the power of a GUI-based admin tool.
PowerShell has a well-integrated command-line utilities/ command for the operation system. It is a simple way to get information about server and is more secure than running VBScript or other scripting languages.
Features of PowerShell
- PowerShell Remoting: The remoting feature allows the execution of PowerShell cmdlets on remote systems that help to manage a set of remote computers from one single machine.
- Background Jobs: PowerShell introduced the concept of background jobs, which run cmdlets and scripts asynchronously on local and remote machines in the background without affecting the interface or interacting with the console.
- Scheduled Job: A scheduled job is like a background job; both jobs are running asynchronously in the background without interrupting the user interface, but the difference is that a background job must be started manually
- Script debugging: As in Visual Studio, you can set breakpoints on lines, columns, functions, variables, and commands.
- Error handling: PowerShell provides error-handling mechanism through the Try{ }, Catch{ }, and Finally { } statements as in .NET languages
PowerShell Cmdlets
A cmdlet is a PowerShell command with a predefined function
Format
- Get — To get something
- Start — To run something
- Out — To output something
- Stop — To stop something that is running
- Set — To define something
- New — To create something
PowerShell Data Types
Data Type Name | Description |
[Array] | Array |
[Bool] | Value is TRUE or FALSE |
[DateTime] | Date and time |
[Guid] | Globally unique 32-byte identifier |
[HashTable] | Hash table, collection of key-value pairs |
[Int32], [Int] | 32-bit integers |
[PsObject] | PowerShell object |
[Regex] | Regular expression |
[ScriptBlock] | PowerShell script block |
[Single], [Float] | Floating point number |
[String] | String |
[Switch] | PowerShell switch parameter |
[TimeSpan] | Time interval |
[XmlDocument] | XML document |
Variables in PowerShell
Simple variable
All variables in PowerShell begin with a US dollar sign ($). The simplest example of this is:
$f = “bar“
Arrays
Array declaration in PowerShell is almost the same as instantiating any other variable,. The items in the array are declared by separating them by commas(,):
$myArrayOfInts = 1,2,3,4
$myArrayOfStrings = “1”,”2″,”3″,”4“
Adding to an Array
Adding to an array is as simple as using the + operator:
$myArrayOfInts = $myArrayOfInts + 5 # now contains 1,2,3,4 & 5!
Combining arrays together
Again this is as simple as using the + operator
$myArrayOfInts = 1,2,3,4
$myOtherArrayOfInts = 5,6,7
$myArrayOfInts = $myArrayOfInts + $myOtherArrayOfInts
Remove-Item Variable:f
- To remove a variable from memory, one can use the Remove-Item cmdlet.
- Another method to remove variable is to use Remove-Variable cmdlet and its alias rv
$var = ‘Some Variable’ #Define variable ‘var’ containing the string ‘Some Variable’
$var
Remove-Variable -Name var
$var
Operators
- An operator is a character that represents an action.
- It tells the compiler/interpreter to perform specific mathematical, relational or logical operation and produce final result.
- PowerShell interprets in a specific way and categorizes accordingly like arithmetic operators strings and other data types.
- Along with the basic operators, PowerShell has several operators that save time and coding effort (e.g.: -like, -match, -replace, etc.).
- Simple comparison operators:
2 -eq 2 # Equal to (==)
2 -ne 4 # Not equal to (!=)
5 -gt 2 # Greater-than (>)
5 -ge 5 # Greater-than or equal to (>=)
5 -lt 10 # Less-than (<) 5
String comparison operators:
“MyString” -like “String” # Match using the wildcard character ()
“MyString” -notlike “Other” # Does not match using the wildcard character ()
“MyString” -match ‘^String$’ # Matches a string using regular expressions
“MyString” -notmatch ‘^Other$’ # Does not match a string using regular expressions
Redirection Operator
- PowerShell redirection operators are using specific characters to specify the output to files Please check the list below:
- – All output
1 – Success output
2 – Errors
3 – Warnings messages
4 – Verbose Output
5 – Debug messages
6 – Informational message
In order to use * , 3 , 4 , 5 you need to have PowerShell 3.0 or above.
Those four types were introduced in Powershell 3.0.
The newer type that has been introduced in PowerShell 5.0 is 6. You need to have PowerShell 5.0 or above to used it.
Assignment Operator
Simple arithmetic:
$var = 1 # Assignment. Sets the value of a variable to the specified value
$var += 2 # Addition. Increases the value of a variable by the specified value
$var -= 1 # Subtraction. Decreases the value of a variable by the specified value
$var *= 2 # Multiplication. Multiplies the value of a variable by the specified value
$var /= 2 # Division. Divides the value of a variable by the specified value
$var %= 2 # Modulus. Divides the value of a variable by the specified value and then
# assigns the remainder (modulus) to the variable
Increment and decrement:
$var++ # Increases the value of a variable, assignable property, or array element by
1 $var– # Decreases the value of a variable, assignable property, or array element by 1
Mixing Operand Types
Note: The type of the left operand dictates the behavior
For Addition
“4” + 2 # Gives “42”
4 + “2” # Gives 6
1,2,3 + “Hello” # Gives 1,2,3,”Hello”
“Hello” + 1,2,3 # Gives “Hello1 2 3”
Redirection Operator
- > Sends output to the specified file
- >> – Appends output to the content of the specified file
- 2> – Sends errors to the specified file.
- 2>> – Appends errors to the content of the specified file
- 2>&1 – Sends errors and success output to the success output stream
- 3> – Sends warnings to the specified file
- 3>> – Appends warnings to the contents of the specified file.
- 3>&1 – Sends warnings and success output to the success output stream
- 4> – Sends verbose output to the specified file
- 4>> – Appends verbose output to the contents of the specified file
- 4>&1 – Sends verbose output and success output to the success output stream
Why should we learn PowerShell?
- All the server products that Microsoft is producing now can be managed through PowerShell.
- Performing the tasks like updating an active directory manually may take hours to complete. By using PowerShell you can complete it using a single command in less time.
- Many of the GUI interfaces that Microsoft has been designing for its various products are front-end interfaces to PowerShell.
- Windows PowerShell Can Reveal “Hidden” Information Not Available in the Admin Center.
- Windows PowerShell is Great at Filtering Data. It lets us do cross-product management.
- PowerShell provides Windows management instruction, enabling administrators to perform administrative tasks on both local and remote Windows systems as well as WS-Management
- Having a solid knowledge of PowerShell scripting will enable us to reduce the time on many administrative functions, without having to purchase and implement third-party tools.
Stay tuned for more updates in PowerShell – Part II, where we will discuss examples on, we can execute it.