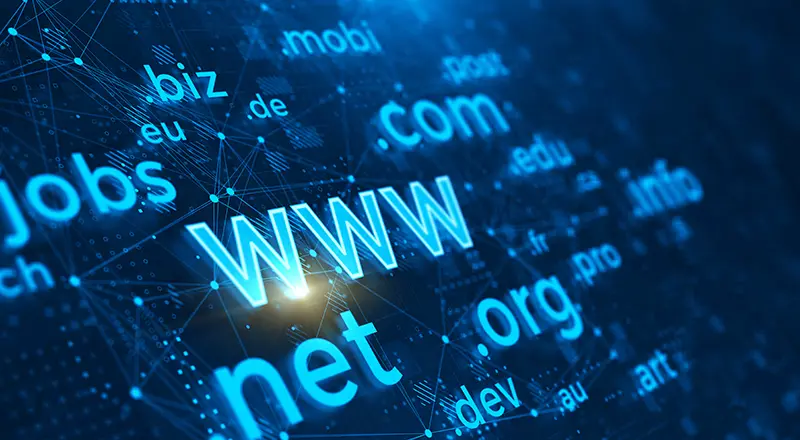
When working with WCF services deployed in SharePoint and ASP.Net, we might have a situation where we need to make an Ajax call from the Web Application outside SharePoint to the WCF service deployed under SharePoint.
This situation points us to the concept of CORS (Cross Origin Support). For a normal WCF service not under SharePoint context, we can make the AJAX calls by using the following ways:
Approach I:
Send data in the HTTP request from Ajax call in the JSON or JSONP format by calling the service methods.
• JSON – works in self-domain
• JSONP – works in cross domain
Approach II:
Create a Proxy of the Service, create a generic handler to call the methods in the service and make Ajax calls to the handler
For a WCF service under Share Point context, the Ajax call cannot be made directly to the service methods as the call made is in a different context. It will give 401 – unauthorized access. Following are the issues to be resolved:
1. Web Application outside the Share Point to get the Share Point context
2. Make a successful Ajax call to the WCF service methods deployed in Share Point
If Approach I is followed as per the code below
paramdata = JSON.stringify ({ ID: 12, Name: Test });
$.ajax({
url: serviceURL,
data: paramdata,
type: “POST”,
dataType: “json”, // “jsonp”
contentType: “application/json; charset=utf-8”,
success: function (data) {
alert(data);
},
error: function (XMLHttpRequest, textStatus, errorThrown) {
alert(textStatus);
document.getElementById(‘pageLoadingContainer’).style.display = “none”;
}
});
for the WCF service deployed in Share Point 2010/2013, we would face the following problems:
– CORS make a pre-flight request to check the server and site to be hit before making the actual calls. This request would fail and gives the following message.

Though we add the header ‘Access-Control-Allow-Origin’ in the Share Point server it doesn’t work as expected. It gives the same error as Share Point doesn’t support the cross origin and is unauthenticated for the user in context. Also Ajax works with WebHTTPBinding, whereas SharePoint service using BasicHTTPBinding.
The above mentioned issues could be resolved by
1. Impersonating the User in the Web Application who has admin access on the Share Point site
2. Create a Proxy and a HTTP module to access the WCF service methods and make successful Ajax calls

This can be accomplished with the following code.
1. In the custom web application web.config, add the Impersonate tag and provide the account credentials which has Admin access on the share point site.
2. Add service reference of the WCF service.
3. Add a generic handler as shown below in the project.
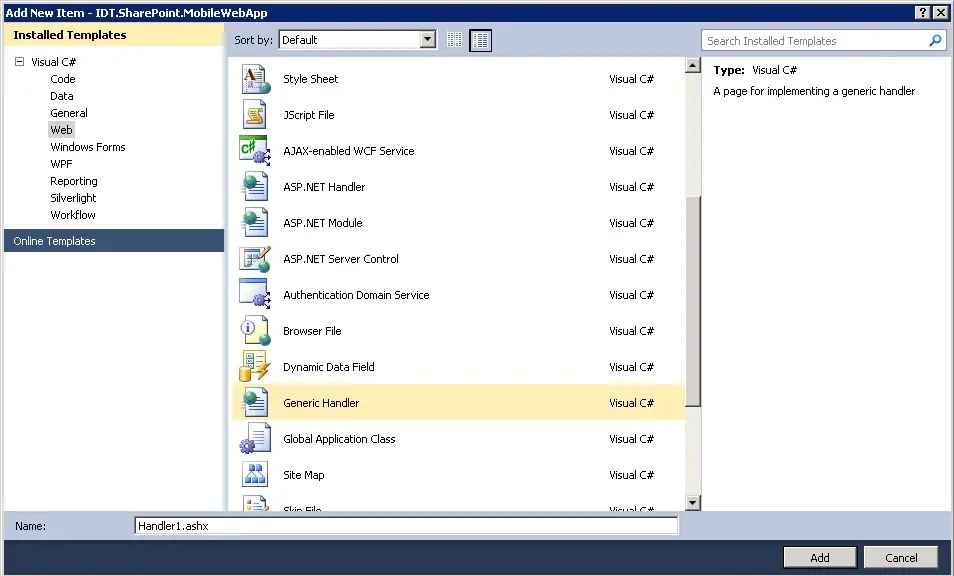
4. In the Handler, call the WCF service method by passing the required parameters as shown below.
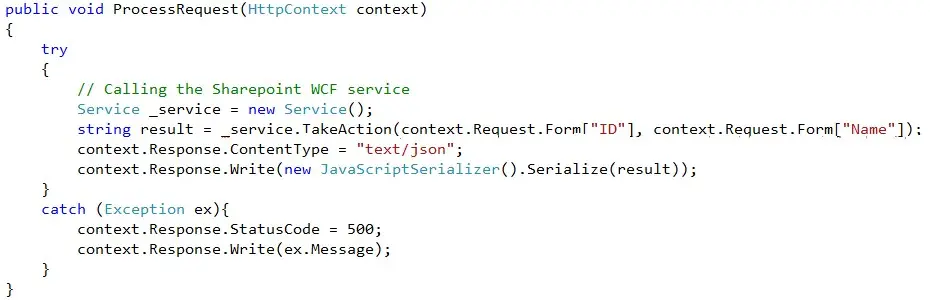
5. Make as Ajax call to the Handler as shown below. The data needs to be specified in the format highlighted in the screenshot below.
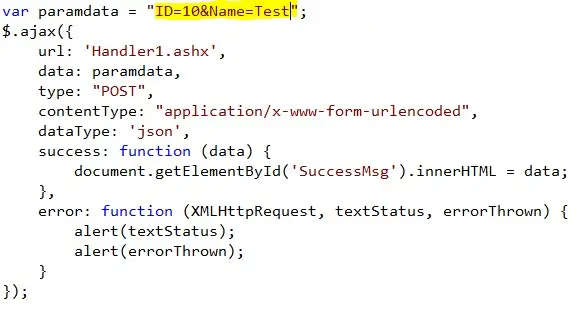
Execute the code now to hit the Success method after the WCF processes the request and displays the success message to the user.