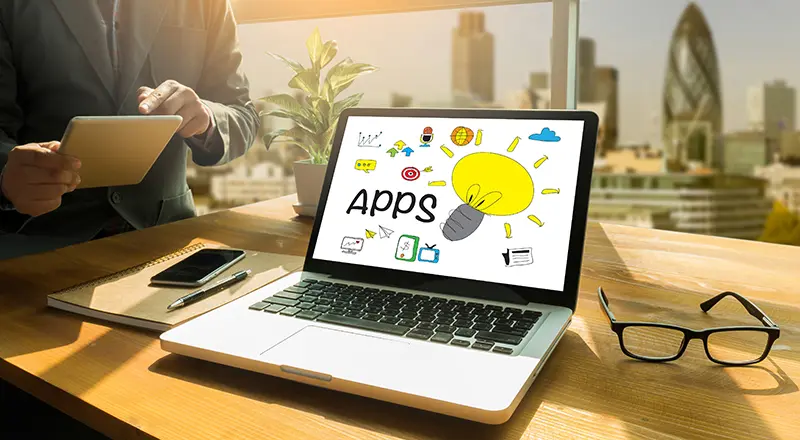
Hybrid App Architecture:
• Web View
• Native Wrapper Integration
Web View
All Web content/app runs inside of Web Views within a Native Wrapper/Container
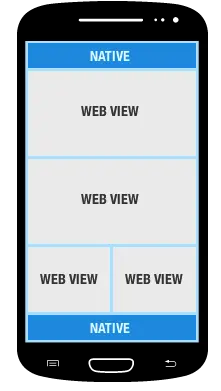
Responsive Web Design: A Web design approach aimed at crafting web pages to provide an optimal viewing experience—easy reading and navigation with a minimum of resizing, panning, and scrolling—across a wide range of devices (from mobile phones to desktop computer monitors). Web content must be Responsive Design to display properly within each defined Web View. A Responsive Design web page displays properly within a the mobile device browser and desktop/notepad browser without any change to the code base.
Multiplatform Support
Leveraging Hybrid App for Multiplatform Support: This requires writing the container in Native mobile language. HTML5 Web components can be reused across all platforms without need to customize.
• iOS: Objective C container + HTML5
• Android: Java Container + HTML5
• Windows: C# Container + HTML5
Leveraging Hybrid App for Cross-platform Support: This requires writing the container in Cross-platform tool only ONCE. HTML5 Web components can be reused across all platforms without need to customize.
• Appcelerator + HTML5 OR Xamarin + HTML5
Multiple Form Factor Support: Responsive Web Design enables support across many form factors without a need to rewrite HTML5 code, namely,
iPhone
• 5: 1136×640
• 4S: 640×960
iPad
• First & second generations: 1024×768
• Third generation: 2048×1536
iPad Mini 1024×768
Android Phones & Tablets
• Small screens: 426dp x 320dp
• Normal screens: 470dp x 320dp
• Large screens: 640dp x 480dp
• Extra-large screens: 960dp x 720dpNative Wrapper Integration
Native: Performance Optimization
Responsive Web Design
• Implement Device-Specific Functionality in Native Code (Speech Recognition, GPS, Accelerometer etc.)
o Faster performance
o Tighter feature integration with the device
o Access to the complete set of device-specific functionality
• Minimize Graphics/Animation on Web Content
o Graphic-intensive pages:Tend to render slowly in HTML, and also require bandwidth to be accessed from remote server
o Animation is optimized within native view
• Optimize Data Access
o Remote content access for dynamically generated HTML pages
o Use Local HTML access for static content
Native/Web View Communication
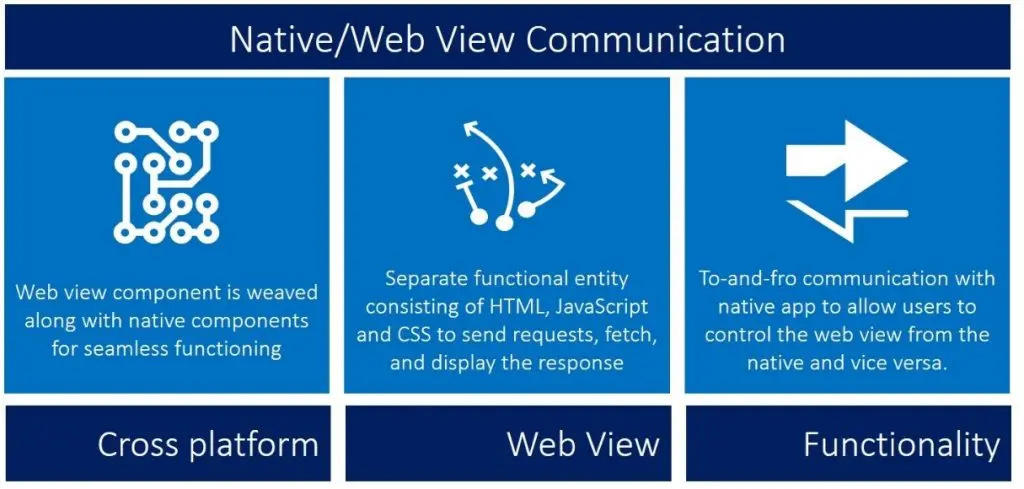
Direct Web View API Invocation
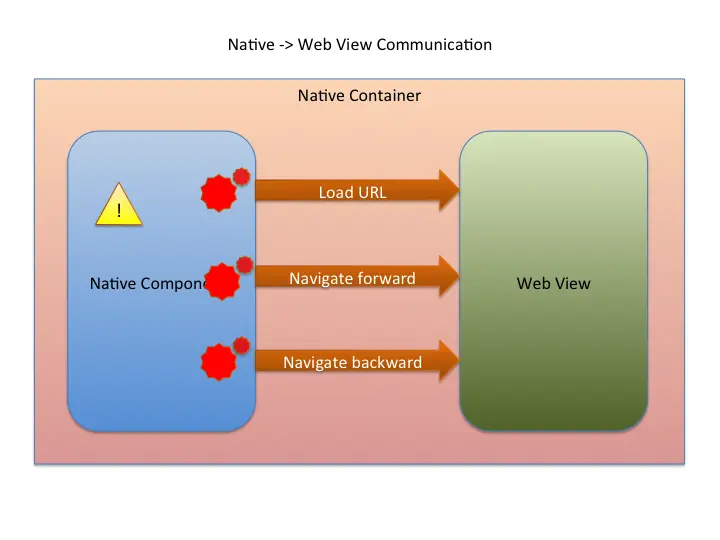
The first aspect of communication between the web view and the native aspect of a hybrid app is about the native part invoking methods on the web view.
Open an URL: The native app invokes a method on the web view component to open and display the contents of a specified URL.
• Lets assume that the web view object is called ‘webview’.
• The native invokes a method on ‘webview’ as such: webview.openURL(‘url_to_open’);
• The web view sends a request, fetches the response and displays the content.
Navigation: The web view component provides API methods to perform navigation while visiting a web site. The native application invokes navigation methods on the web view
• webview.goForward(); – Allows the user to navigate forward in history
• webview.goBackward(); – Allows the user to navigate backward in history
• webview.canGoForward(); – Allows the user to test if forward navigation can be done
• webview.canGoBack(); – Allows the user to test if backward navigation can be done
Web View to Native: Web View Event Captures
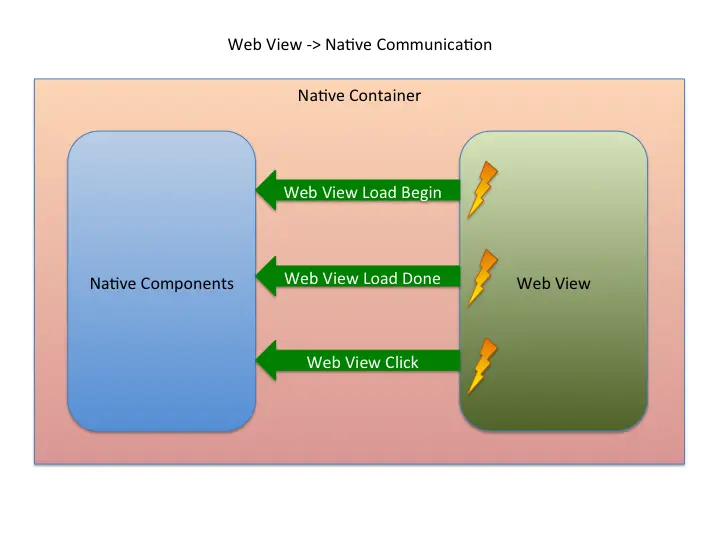
There are certain events generated from the web view that indicate the occurrence of an activity on the web view. These include:
• Web page beginning to load
• Web page loading done
• Single tap/Double tap events on web view
• Swipe event on web view
• Click event on web view
When these events are fired from the web view, the native app can capture these by means of event handlers so that an appropriate action can be executed. Typically, this is done on Appcelerator Studio or Titanium Studio using event listeners
• webview.addEventListener(‘load’, function(){
//event handler code goes here
});
On native platforms like iOS, this is done using delegate methods.
JavaScript Injections
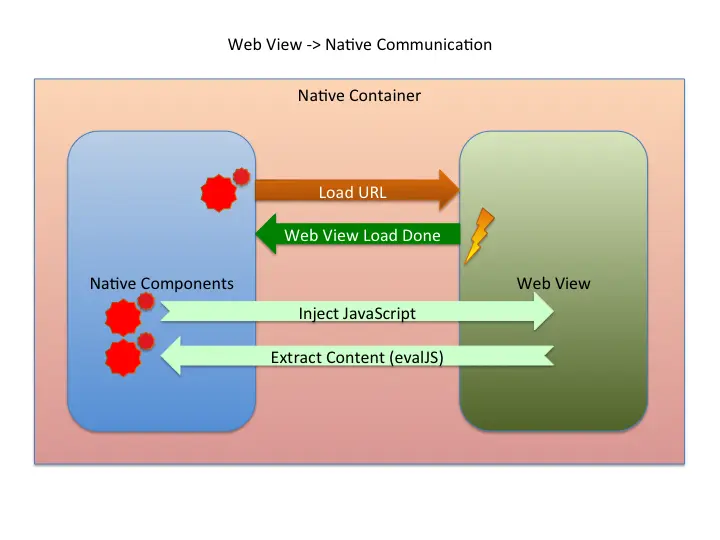
• The native app can communicate with the web view to send messages using the first methodology we talked about earlier.
• However, there are scenarios when the native app needs to fetch or send custom data from/to the web view respectively.
• This is because of the fact that the web page displayed in the web view usually consists of HTML and JavaScript.
• In order to change certain behavior of the web page being displayed in the web view, or to fetch data from the web view, which otherwise won’t be accessible directly, the native app needs to be able to send or pull data pertaining to the web view’s execution environment.
• Generally, the web view’s execution environment runs JavaScript, which has its own scope, variables, methods, event listeners etc.
• Attempting to solve the issues outlined above requires injection of custom JavaScript into the web view’s execution environment.
• This may also involve running a JavaScript code against the web view to fetch certain data pertaining to either the DOM or the web view’s JavaScript scope.
• On Appcelerator Studio, this is achieved by invoking the method call ‘evalJS’ on the web view object.
• Using ‘evalJS’, JavaScript code can be injected to alter the web page’s behavior, or the native app can send/fetch custom data to/from the web page.