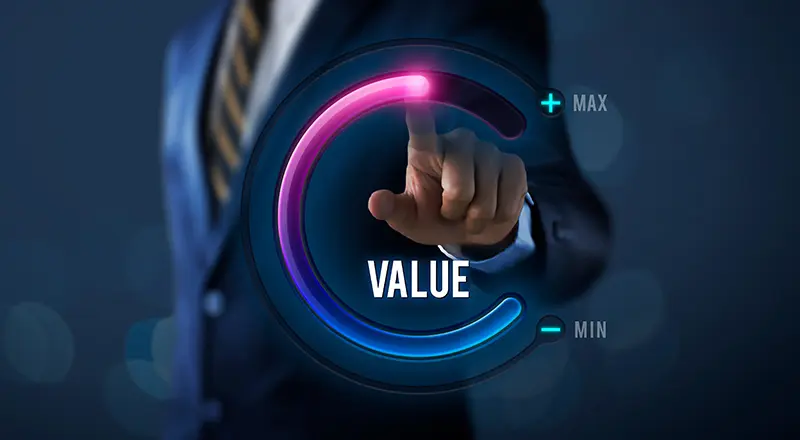
In many applications, there might be a situation where it is required to map different types of objects. Many a times applications don’t expose the actual database entity to the world. It always prefers to give the Data Transfer Object (DTO) rather than the actual object. Also while writing some business logic there may be a need to move data from temp tables to actual tables. In these situations, Automapper gives opportunity to avoid writing dirty code (object to object mapping).
• Install “AutoMapper” using package manager console.

Objects with Same Properties:
1. Below are two classes one actual and the other a DTO class.
public class Order
{
public string UserName { get; set; }
public string Item { get; set; }
public decimal Price { get; set; }
public int Quantity { get; set; }
}
public class OrderDTO
{
public string Item { get; set; }
public string UserName { get; set; }
public decimal Price { get; set; }
public int Quantity { get; set; }
}
2. Automapper requires to configure the mapping of the objects before it can be used
For example, in this sample application where the conversion of Order object to Order DTO is being done, first configure the same in starting of the application or before calling the map method.
Mapper.CreateMap<Order, OrderDTO>();
3. Create an Order object with static data (In real time this data might come from database).
Order orderObj = new Order()
{
Item = “Item name”,
Price = 5,
Quantity = 1,
UserName = “Username”
};
4. Call the map method to get the converted object as shown below.
OrderDTO _orderDTO = Mapper.Map<Order, OrderDTO>(orderObj);
5. Automapper returns the mapped object.
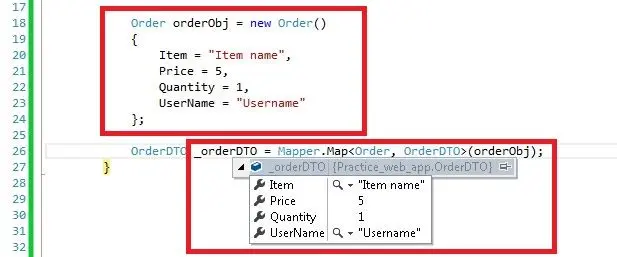
Objects with Different Properties:
- Generally, there may be a case where one object is present as a property in another object. While exposing DTO’s, preferably it’s a good practice to give all the required properties in one object rather than in many objects.
For example order object contains the user object and that contains the name of the user. Here are the objects:
publicclassUser
{
publicstring Name { get; set; }
}
publicclassOrder
{
publicUser User { get; set; }
publicstring Item { get; set; }
publicdecimal Price { get; set; }
publicint Quantity { get; set; }
}
- Update the sample code as mentioned below and run the application. The UserName in the DTO object is assigned with the value of the entity. This is because the entityname concatenated with the propertyname (entityname + propertyname) matches the DTO property name.
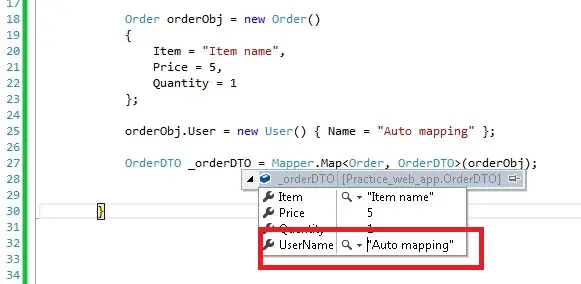